AutoComplete.el : Python Code Completion in Emacs
January 21, 2009 at 10:01 PM | categories: python, emacs, linux | View Comments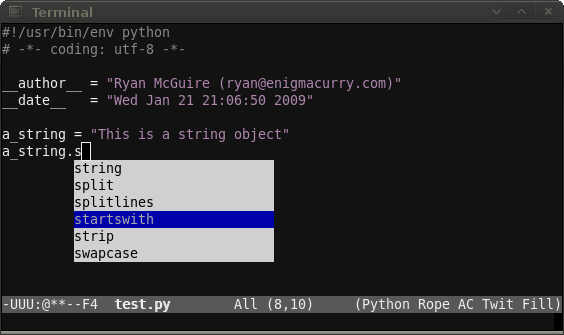
A friend of mine and I like to show off to each other little Emacs tips and tricks we learn. Today, he introduced to me the very cool AutoComplete.el package. AutoComplete.el is intriguing to me because, unlike ropemacs mode which I've blogged about before, the completions AutoComplete.el provides are inline with your code in a dropdown box instead of in a seperate window (windows in Emacs are what most people call frames). However, AutoComplete is only a completion framework, it doesn't know anything about Python. Instead, it allows the user to plug into it, feeding it whatever sorts of intelligent completion you want, including Rope.
Setup
The two most important completions I want to integrate into it are Rope and yasnippet. AutoComplete can handle both of them nicely. You'll need to install the very latest development version (as of December '08) of Rope, Ropemacs and Ropemode:
sudo apt-get install mercurial mkdir /tmp/rope && cd /tmp/rope hg clone http://bitbucket.org/agr/rope hg clone http://bitbucket.org/agr/ropemacs hg clone http://bitbucket.org/agr/ropemode sudo easy_install rope ln -s ../ropemode/ropemode ropemacs/ sudo easy_install ropemacs
You'll also need to install Pymacs and Yasnippet if you haven't already:
mkdir -p ~/.emacs.d/vendor && cd ~/.emacs.d/vendor wget http://pymacs.progiciels-bpi.ca/archives/Pymacs-0.23.tar.gz tar xfv Pymacs-0.23.tar.gz cd Pymacs-0.23 make sudo easy_install . cd ~/.emacs.d/vendor wget http://yasnippet.googlecode.com/files/yasnippet-0.5.9.tar.bz2 tar xfv yasnippet-0.5.9.tar.bz2 cd ~/.emacs.d ln -s vendor/yasnippet-0.5.9/snippets/ .
Make sure Pymacs and Yasnippet get into your load path, in your .emacs:
(add-to-list 'load-path "~/.emacs.d/vendor") (progn (cd "~/.emacs.d/vendor") (normal-top-level-add-subdirs-to-load-path))
Install AutoComplete.el 0.1.0 (0.2.0 is now out, and is not compatible with this post, I'll try and update later):
cd ~/.emacs.d/vendor wget http://www.emacswiki.org/emacs/?action=browse;id=auto-complete.el;raw=1;revision=5
Now to add some more elisp to your .emacs somewhere:
(require 'python) (require 'auto-complete) (require 'yasnippet) (autoload 'python-mode "python-mode" "Python Mode." t) (add-to-list 'auto-mode-alist '("\\.py\\'" . python-mode)) (add-to-list 'interpreter-mode-alist '("python" . python-mode)) ;; Initialize Pymacs (autoload 'pymacs-apply "pymacs") (autoload 'pymacs-call "pymacs") (autoload 'pymacs-eval "pymacs" nil t) (autoload 'pymacs-exec "pymacs" nil t) (autoload 'pymacs-load "pymacs" nil t) ;; Initialize Rope (pymacs-load "ropemacs" "rope-") (setq ropemacs-enable-autoimport t) ;; Initialize Yasnippet ;Don't map TAB to yasnippet ;In fact, set it to something we'll never use because ;we'll only ever trigger it indirectly. (setq yas/trigger-key (kbd "C-c <kp-multiply>")) (yas/initialize) (yas/load-directory "~/.emacs.d/snippets") ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; ;;; Auto-completion ;;; Integrates: ;;; 1) Rope ;;; 2) Yasnippet ;;; all with AutoComplete.el ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; (defun prefix-list-elements (list prefix) (let (value) (nreverse (dolist (element list value) (setq value (cons (format "%s%s" prefix element) value)))))) (defvar ac-source-rope '((candidates . (lambda () (prefix-list-elements (rope-completions) ac-target)))) "Source for Rope") (defun ac-python-find () "Python `ac-find-function'." (require 'thingatpt) (let ((symbol (car-safe (bounds-of-thing-at-point 'symbol)))) (if (null symbol) (if (string= "." (buffer-substring (- (point) 1) (point))) (point) nil) symbol))) (defun ac-python-candidate () "Python `ac-candidates-function'" (let (candidates) (dolist (source ac-sources) (if (symbolp source) (setq source (symbol-value source))) (let* ((ac-limit (or (cdr-safe (assq 'limit source)) ac-limit)) (requires (cdr-safe (assq 'requires source))) cand) (if (or (null requires) (>= (length ac-target) requires)) (setq cand (delq nil (mapcar (lambda (candidate) (propertize candidate 'source source)) (funcall (cdr (assq 'candidates source))))))) (if (and (> ac-limit 1) (> (length cand) ac-limit)) (setcdr (nthcdr (1- ac-limit) cand) nil)) (setq candidates (append candidates cand)))) (delete-dups candidates))) (add-hook 'python-mode-hook (lambda () (auto-complete-mode 1) (set (make-local-variable 'ac-sources) (append ac-sources '(ac-source-rope) '(ac-source-yasnippet))) (set (make-local-variable 'ac-find-function) 'ac-python-find) (set (make-local-variable 'ac-candidate-function) 'ac-python-candidate) (set (make-local-variable 'ac-auto-start) nil))) ;;Ryan's python specific tab completion (defun ryan-python-tab () ; Try the following: ; 1) Do a yasnippet expansion ; 2) Do a Rope code completion ; 3) Do an indent (interactive) (if (eql (ac-start) 0) (indent-for-tab-command))) (defadvice ac-start (before advice-turn-on-auto-start activate) (set (make-local-variable 'ac-auto-start) t)) (defadvice ac-cleanup (after advice-turn-off-auto-start activate) (set (make-local-variable 'ac-auto-start) nil)) (define-key python-mode-map "\t" 'ryan-python-tab) ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;; ;;; End Auto Completion ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
Github
These changes are also applied to my Emacs environment on Github. I'm continuously trying to improve my emacs setup, so if you're reading this a few months/years after this post was made, you may want to check there for improvements.
Update Jan 30 2009: I made some modifications to the tab complete order, a regular indent is applied first before autocompletion. Autocompletion is also not applied unless you are at the end of a word. This is useful when you press Tab at the beginning of a line to indent/outdent.
Usage
Once you got everything setup, usage is real easy:
- Open up a python (.py) file
- Press TAB when you want to use code completion or to insert a snippet.
The first time you attempt to use code completion you'll be prompted to enter the root of your project directory.
Future
It wasn't too long ago when Python code completion inside Emacs was just a pipedream. So now that we have it, let's make some more pipedreams: I'd like to popup some nice contextual help for method arguments as well as python documentation for the current method/class being completed.
The possibilities are pretty much endless. I love Emacs.